I created a ‘Guess My Number’ game using JavaScript to explore DOM manipulation and CSS styling directly through JS. This project was a hands-on challenge where I implemented interactivity and logic, gaining a deeper understanding of how JavaScript interacts with HTML and CSS. The game lets users guess a randomly generated number, provides feedback, and visually adjusts the interface dynamically. It was challenging, but it was a great way to explore the ins and outs of JavaScript!
Complete Code for the Guess My Number Game:
HTML Code:
code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Guess My Number</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #222;
color: #eee;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
text-align: center;
}
.number {
font-size: 3rem;
background: #eee;
color: #222;
width: 3rem;
margin: 1rem auto;
}
.message {
margin: 1rem 0;
}
.input-container {
margin: 1rem 0;
}
.input-container input {
width: 50px;
font-size: 1rem;
}
.btn {
padding: 0.5rem 1rem;
font-size: 1rem;
cursor: pointer;
margin: 0.5rem;
}
.hidden {
display: none;
}
</style>
</head>
<body>
<div class="container">
<h1>Guess My Number!</h1>
<div class="number hidden">?</div>
<p class="message">Start guessing...</p>
<div class="input-container">
<input type="number" class="guess" min="1" max="20" />
<button class="btn check">Check!</button>
</div>
<p class="score">Score: <span>20</span></p>
<p class="highscore">Highscore: <span>0</span></p>
<button class="btn again">Play Again</button>
</div>
<script src="script.js"></script>
</body>
</html>
JavaScript Code:
code'use strict';
// Variables for game logic
let secretNumber = Math.trunc(Math.random() * 20) + 1;
let score = 20;
let highscore = 0;
// Functions for UI updates
const displayMessage = function (message) {
document.querySelector('.message').textContent = message;
};
const setBackgroundColor = function (color) {
document.querySelector('body').style.backgroundColor = color;
};
// Game functionality
document.querySelector('.check').addEventListener('click', function () {
const guess = Number(document.querySelector('.guess').value);
// When no input
if (!guess) {
displayMessage('⛔ No number!');
// When player wins
} else if (guess === secretNumber) {
displayMessage('🎉 Correct Number!');
document.querySelector('.number').textContent = secretNumber;
setBackgroundColor('#60b347');
document.querySelector('.number').style.width = '30rem';
// Update highscore
if (score > highscore) {
highscore = score;
document.querySelector('.highscore span').textContent = highscore;
}
// When guess is wrong
} else if (guess !== secretNumber) {
if (score > 1) {
displayMessage(guess > secretNumber ? '📈 Too high!' : '📉 Too low!');
score--;
document.querySelector('.score span').textContent = score;
} else {
displayMessage('💥 You lost the game!');
document.querySelector('.score span').textContent = 0;
}
}
});
// Reset game functionality
document.querySelector('.again').addEventListener('click', function () {
score = 20;
secretNumber = Math.trunc(Math.random() * 20) + 1;
displayMessage('Start guessing...');
document.querySelector('.score span').textContent = score;
document.querySelector('.number').textContent = '?';
document.querySelector('.guess').value = '';
setBackgroundColor('#222');
document.querySelector('.number').style.width = '15rem';
});
See the result output click here Run Compiler after open this file in new tab than you will click just Run button the game to the starting showing result
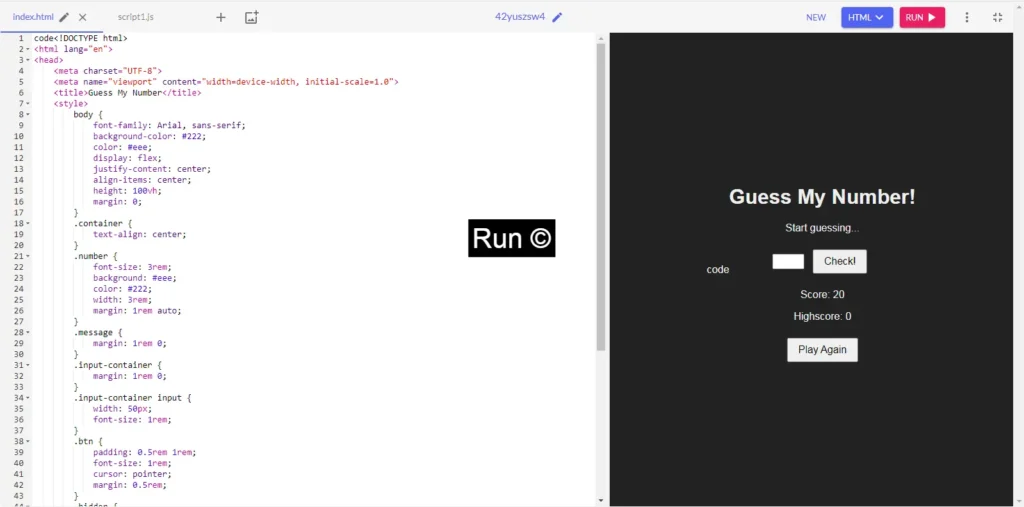
How the Game Works:
- Random Number Generation:
- A secret number between 1 and 20 is generated.
- User Input:
- The user inputs their guess via the text box.
- Game Logic:
- If the guess is correct, the game announces a win, updates the high score, and changes the background to green.
- If the guess is too high or too low, feedback is displayed, and the score decreases.
- Reset Game:
- Clicking “Play Again” resets the game to its original state.
Key Learning Points:
- DOM Manipulation:
document.querySelector()
to select elements.textContent
andstyle
to update content and appearance dynamically.
- JavaScript Logic:
- Conditional statements for feedback.
- Use of event listeners for button clicks.
- CSS Integration:
- Dynamically changing styles using JavaScript for a seamless user experience.
This game taught me how to blend JavaScript and CSS for interactive projects and provided a strong understanding of real-world JS application. Let me know if you’d like to add more features!