Hi, I’m here to explain this HTML and CSS code that creates a sleek, responsive nutrition label, just like what you’d see on food packaging. The label is styled and structured to display nutritional information clearly and aesthetically. Let me walk you through its components and functionality.
Explaining the Code:
HTML (Structure):
The HTML code defines the structure of the nutrition label:
Document Type Declaration:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Nutrition Label</title>
<link href="https://fonts.googleapis.com/css?family=Open+Sans: 400, 700, 800" rel="stylesheet">
<link href="./styles.css" rel="stylesheet">
</head>
<body>
<div class="label">
<header>
<h1 class="bold">Nutrition Facts</h1>
<div class="divider"></div>
<p>8 servings per container</p>
<p class="bold">Serving size <span>2/3 cup (55g)</span></p>
</header>
<div class="divider large"></div>
<div class="calories-info">
<div class="left-container">
<h2 class="bold small-text">Amount per serving</h2>
<p>Calories</p>
</div>
<span>230</span>
</div>
<div class="divider medium"></div>
<div class="daily-value small-text">
<p class="bold right no-divider">% Daily Value *</p>
<div class="divider"></div>
<p><span><span class="bold">Total Fat</span> 8g</span> <span class="bold">10%</span></p>
<p class="indent no-divider">Saturated Fat 1g <span class="bold">5%</span></p>
<div class="divider"></div>
<p class="indent no-divider"><span><i>Trans</i> Fat 0g</span></p>
<div class="divider"></div>
<p><span><span class="bold">Cholesterol</span> 0mg</span> <span class="bold">0%</span></p>
<p><span><span class="bold">Sodium</span> 160mg</span> <span class="bold">7%</span></p>
<p><span><span class="bold">Total Carbohydrate</span> 37g</span> <span class="bold">13%</span></p>
<p class="indent no-divider">Dietary Fiber 4g</p>
<div class="divider"></div>
<p class="indent no-divider">Total Sugars 12g</p>
<div class="divider double-indent"></div>
<p class="double-indent no-divider">Includes 10g Added Sugars <span class="bold">20%</span></p>
<div class="divider"></div>
<p class="no-divider"><span><span class="bold">Protein</span> 3g</span></p>
<div class="divider large"></div>
<div class="divider large"></div>
<p>Vitamin D 2mcg <span>10%</span></p>
<p>Calcium 260mg <span>20%</span></p>
<p>Iron 8mg <span>45%</span></p>
<p class="no-divider">Potassium 235mg <span>6%</span></p>
</div>
<div class="divider medium"></div>
<p class="note">* The % Daily Value (DV) tells you how much a nutrient in a serving of food contributes to a daily diet. 2,000 calories a day is used for general nutrition advice.</p>
</div>
</body>
</html>
This ensures the browser interprets the document as HTML5.
Basic Setup:
Specifies the language as English for accessibility.
- Head Section:
- Includes the character encoding (
UTF-8
), ensuring the document supports various characters. - Links Google Fonts for the
Open Sans
font and a custom CSS file for styling.
- Includes the character encoding (
- Body Section:
- Contains a
div
with the classlabel
as the container for the nutrition label. - Nested within the
label
are various sections:- Header: Displays “Nutrition Facts” as a heading, with serving details.
- Calories Info: Highlights the calories per serving in a bold and prominent style.
- Daily Value Section: Lists nutrient values and their percentages.
- Notes: Explains the % Daily Value calculation.
- Contains a
CSS (Styling):
The CSS brings life to the label with structured design and color schemes:
*{
box-sizing: border-box;
}
html {
font-size: 16px;
}
body {
font-family: 'Open Sans', sans-serif;
}
.label {
border: 2px solid rgb(94, 3, 3);
border-radius: 3px;
width: 270px;
margin: 20px auto;
margin-top: 100px;
padding: 0 7px;
background: rgb(236, 237, 239);
}
header h1 {
text-align: center;
margin: -4px 0;
letter-spacing: 0.15px
}
p {
margin: 0;
display: flex;
justify-content: space-between;
}
.divider {
border-bottom: 1px solid #888989;
margin: 2px 0;
}
.bold {
font-weight: 800;
}
.large {
height: 10px;
}
.large,
.medium {
background-color: black;
border: 0;
}
.medium {
height: 5px;
}
.small-text {
font-size: 0.85rem;
}
.calories-info {
display: flex;
justify-content: space-between;
align-items: flex-end;
}
.calories-info h2 {
margin: 0;
}
.left-container p {
margin: 5px 2px;
font-size: 2em;
font-weight: 700;
}
.calories-info span {
margin: -7px 2px;
font-size: 2.4em;
font-weight: 700;
}
.right {
justify-content: flex-end;
}
.indent {
margin-left: 1em;
}
.double-indent {
margin-left: 2em;
}
.daily-value p:not(.no-divider) {
border-bottom: 1px solid #888989;
}
.note {
font-size: 0.6rem;
margin-top: 5px;
margin-bottom: 5px;
margin-left: 0;
margin-right: 0;
padding: 8px;
text-indent: -8px;
}
body{
background:
rgb(170, 227, 255);
}
- Global Styles:
- All elements (
*
) usebox-sizing: border-box
for predictable sizing. - The default font size is set to
16px
, making it easier to use rem-based units.
- All elements (
- Body and Container:
- The label is styled as a centered card with a solid border, rounded corners, and a neutral background color.
- Typography:
- Bold text (
font-weight: 800
) for emphasis on important details. - Scaled fonts (
small-text
,large
,medium
) ensure hierarchical clarity.
- Bold text (
- Dividers:
- Horizontal lines (
.divider
) separate sections, with variations like.large
and.medium
for thickness.
- Horizontal lines (
- Alignment:
- Flexbox ensures proper alignment and spacing of items, especially in calorie and nutrient sections.
- Background:
- The background is a soothing blue, enhancing readability and aesthetics.
Final Outcome Result:
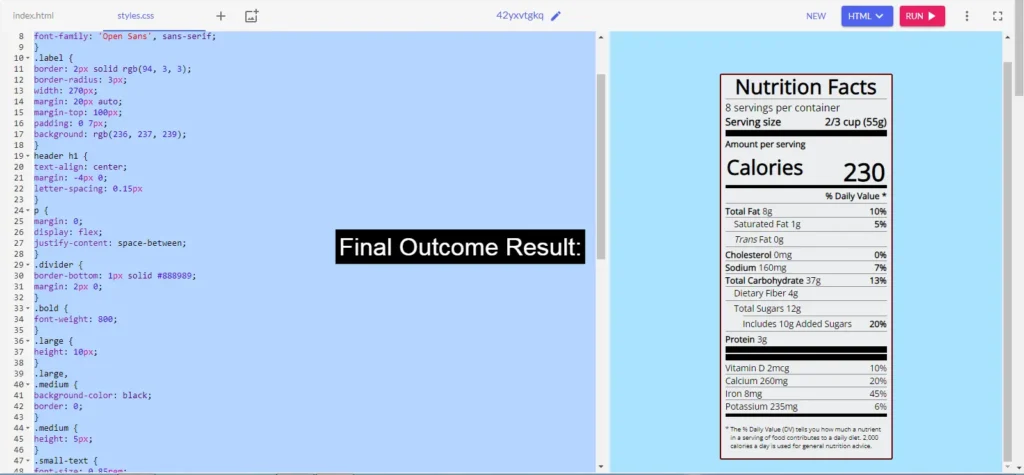
When rendered, this code creates a clean, visually appealing nutrition label with clear separation of information. The design adheres to accessibility principles and is easy to adapt for various devices or additional styling.